Object-Oriented Programming (OOP) is a key concept in software development. It helps programmers create organized and efficient code.
C++ is one of the most popular languages that supports OOP. Many developers use C++ to build a wide range of applications. Understanding OOP in C++ can make programming easier and more effective. It allows you to think about real-world objects and their interactions.
This approach improves code reusability and makes it simpler to manage complex systems. In this blog post, we will explore the main ideas of OOP in C++. You will learn about its key features, such as classes, objects, inheritance, and polymorphism. Get ready to dive into the world of C++ and discover how OOP can enhance your programming skills.
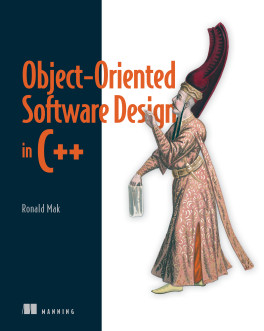
Credit: www.manning.com
The Pillars Of Oop In C++
Object-Oriented Programming (OOP) in C++ is built on four main pillars. These pillars are essential for creating efficient and manageable code. They help programmers design robust applications. Understanding these concepts is crucial for mastering C++.
Encapsulation: Data & Function Cohesion
Encapsulation means bundling data with methods that work on that data. It keeps data safe from outside interference. This is done using classes. A class combines attributes and methods.
Key benefits of encapsulation include:
- Data hiding: Protects sensitive data.
- Modularity: Breaks code into smaller parts.
- Maintainability: Easier to update and fix.
Here is a simple example of encapsulation:
class Box {
private:
int width;
public:
void setWidth(int w) {
width = w;
}
int getWidth() {
return width;
}
};
Abstraction: Simplifying Complexity
Abstraction helps reduce complexity. It allows you to focus on essential features. Unnecessary details are hidden from the user. This makes the code easier to understand.
Abstraction is achieved through:
- Abstract classes: Classes that cannot be instantiated.
- Interfaces: Define methods without implementation.
Example of an abstract class:
class Animal {
public:
virtual void sound() = 0; // Pure virtual function
};
Inheritance: Building On Base Classes
Inheritance allows one class to inherit properties from another. This creates a hierarchy. It promotes code reuse and reduces redundancy.
Types of inheritance include:
- Single Inheritance: One base class.
- Multiple Inheritance: More than one base class.
Example of inheritance:
class Dog : public Animal {
public:
void sound() {
cout << "Bark" << endl;
}
};
Polymorphism: Flexible Interfaces
Polymorphism enables objects to be treated as instances of their parent class. It allows methods to do different things based on the object. This provides flexibility in code.
Types of polymorphism include:
- Compile-time polymorphism: Achieved through function overloading.
- Runtime polymorphism: Achieved through function overriding.
Example of polymorphism:
void makeSound(Animal &a) {
a.sound();
}
Setting Up The C++ Environment
Setting up the C++ environment is the first step to coding. It involves selecting the right tools. You need an IDE, a compiler, and a linker. These tools help you write and run your C++ programs.
Let’s explore how to set up everything you need to start coding in C++.
Choosing An Ide
An Integrated Development Environment (IDE) makes coding easier. It combines a code editor, compiler, and debugger. Popular IDEs for C++ include Code::Blocks, Eclipse, and Visual Studio. Each IDE has its own features.
Choose one based on your needs. Beginners might prefer simpler IDEs. More advanced users may choose feature-rich options. Install the IDE of your choice. Follow the setup instructions provided.
Compilers And Linkers
A C++ compiler translates your code into machine language. This allows your computer to run it. Common compilers include GCC and Clang. Linkers combine your compiled code with libraries.
Make sure your IDE includes a compiler. Most do, but check the documentation. Install any additional tools if necessary. This ensures everything works correctly.
Hello World: Your First C++ Oop Program
Now it’s time to write your first C++ program. Open your IDE and create a new project. Name it “HelloWorld.” In the main file, type the following code:
#include using namespace std; int main() { cout << "Hello, World!" << endl; return 0; }
This code displays “Hello, World!” on the screen. Save your file. Click the “Run” button in your IDE. You should see the message. Congratulations! You’ve just written your first C++ OOP program.
Classes And Objects: The Basics
Object-Oriented Programming (OOP) in C++ uses classes and objects. These are the building blocks of OOP. A class is like a blueprint. An object is an instance of that blueprint. Understanding classes and objects is key to mastering OOP.
Defining Classes
A class defines a type. It groups data and functions that work on that data. Here is how to define a class in C++:
class Car {
public:
string brand;
int year;
void display() {
cout << "Brand: " << brand << ", Year: " << year << endl;
}
};
In this example:
- Car is the class name.
- brand and year are attributes.
- display() is a method that shows car details.
Creating Objects
Creating an object is simple. Use the class name followed by the object name. Here is an example:
Car myCar;
myCar.brand = "Toyota";
myCar.year = 2020;
myCar.display();
In this code:
- myCar is an object of the class Car.
- We assign values to brand and year.
- Finally, we call the display() method.
Accessing Members
Accessing members of a class is straightforward. Use the dot operator (.) to access attributes and methods. Here are some points to remember:
Member Type | Access Method |
---|---|
Attribute | objectName.attributeName |
Method | objectName.methodName() |
For example:
cout << myCar.brand; // Accessing attribute
myCar.display(); // Calling method
Classes and objects help organize code. They promote reuse and maintainability. Understanding these concepts is essential for effective programming in C++.
Constructor And Destructor Deep Dive
Understanding constructors and destructors is essential in C++. These special functions help manage object lifecycles. They ensure proper resource allocation and deallocation. Let’s explore their key features.
Purpose Of Constructors
Constructors initialize objects when they are created. They set default values for attributes. This ensures that objects start in a valid state. Constructors have the same name as the class. They do not return any value. This makes them unique among functions.
There are different types of constructors. The default constructor takes no parameters. The parameterized constructor takes arguments. This allows for customization during object creation. Constructors are crucial for building reliable applications.
Destructor Functionality
Destructors are the opposite of constructors. They clean up resources before an object is destroyed. They help prevent memory leaks. Destructors have the same name as the class but with a tilde (~) prefix. Like constructors, destructors do not return any value.
Destructors are called automatically when an object goes out of scope. This automatic call helps maintain memory integrity. Proper use of destructors is vital for effective resource management.
Constructor Overloading
Constructor overloading allows multiple constructors in a class. Each constructor can have different parameters. This adds flexibility in object creation. You can create objects in various ways based on needs.
Overloaded constructors share the same name. The compiler distinguishes them by their parameter lists. This feature enhances code readability and usability. Using constructor overloading can simplify your code.
Operator Overloading In C++
Operator Overloading in C++ allows programmers to redefine how operators work. This feature enhances code readability and usability. By using operator overloading, you can treat objects like basic data types. This makes your code easier to understand and maintain.
Basics Of Operator Overloading
Operator overloading lets you define custom behavior for operators. This includes symbols like +, -, , and /. Here are some key points:
- Operators can be overloaded for user-defined types.
- It improves clarity in code.
- Operators can be overloaded as member functions or friend functions.
To overload an operator, you must define a function. This function specifies what the operator should do. For example, to overload the + operator, you create a function like this:
Complex operator+(const Complex &other);
In this case, the function will add two Complex objects together.
Overloading Unary Operators
Unary operators work on one operand. Common examples are increment (++) and decrement (–). Here’s how to overload a unary operator:
class Counter {
public:
int value;
Counter(int v) : value(v) {}
// Overloading the ++ operator
Counter operator++() {
return Counter(++value);
}
};
This example shows how the ++ operator increases the value of a Counter object. Each time you call it, the value increases by one.
Overloading Binary Operators
Binary operators work on two operands. Examples include addition (+) and subtraction (-). To overload a binary operator:
class Complex {
public:
float real, imag;
Complex(float r, float i) : real(r), imag(i) {}
// Overloading the + operator
Complex operator+(const Complex &other) {
return Complex(real + other.real, imag + other.imag);
}
};
This code allows you to add two Complex numbers. The overloaded + operator returns a new Complex number.
Here’s a quick comparison of unary and binary operator overloading:
Operator Type | Example | Function Signature |
---|---|---|
Unary | ++ | Counter operator++(); |
Binary | + | Complex operator+(const Complex &other); |
Operator overloading in C++ enhances code clarity. It allows objects to behave like basic data types. Use it wisely to improve your programming experience.
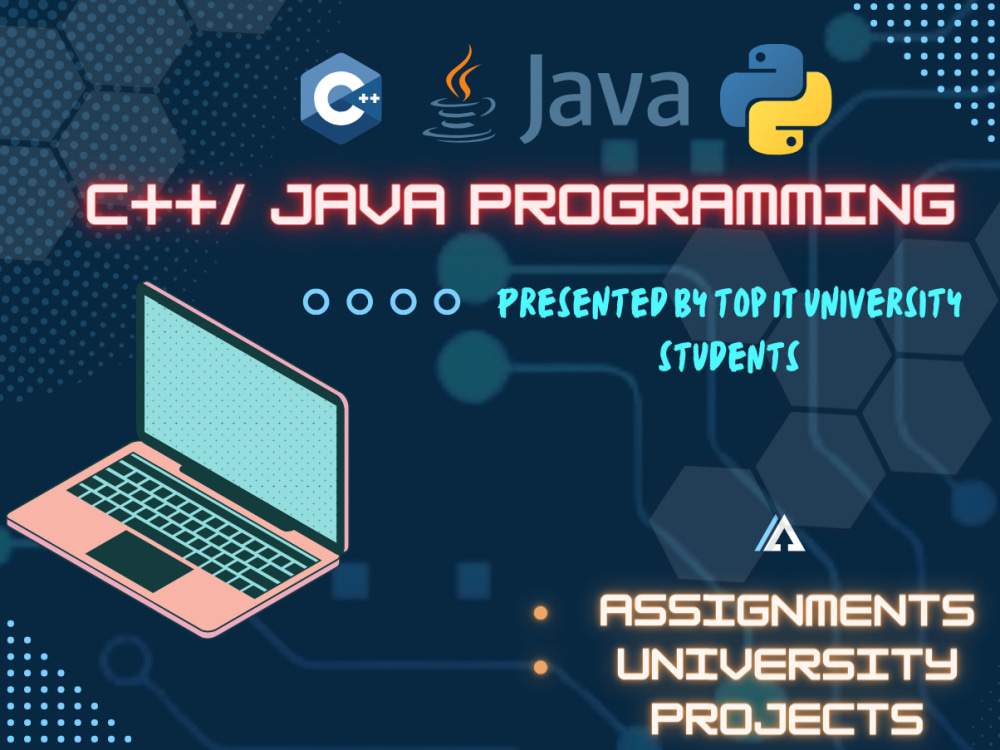
Credit: www.upwork.com
Inheritance Explored
Inheritance is a key concept in Object-Oriented Programming (OOP). It allows one class to inherit properties and methods from another. This helps in code reusability and reduces redundancy. In C++, inheritance creates a hierarchy of classes. Let’s dive into the different types of inheritance and understand their significance.
Types Of Inheritance
In C++, there are several types of inheritance. Each type serves a different purpose. Here are the main types:
Type | Description |
---|---|
Single Inheritance | One class inherits from another class. |
Multiple Inheritance | One class inherits from multiple classes. |
Multilevel Inheritance | A class inherits from a derived class. |
Hierarchical Inheritance | Multiple classes inherit from a single-parent class. |
Hybrid Inheritance | A combination of two or more types of inheritance. |
Protected Members And Accessibility
Protected members play a vital role in inheritance. They are accessible in derived classes but not outside them. This allows controlled access to class data.
Here are the key points about protected members:
- Protected members are declared using the
protected
keyword. - Only derived classes can access protected members.
- They help maintain encapsulation.
Example:
class Base {
protected:
int value;
};
class Derived : public Base {
public:
void setValue(int v) {
value = v; // Accessible here
}
};
Inheritance Vs Composition
Inheritance and composition are two important concepts in OOP. Both have their uses and advantages.
Here’s a quick comparison:
Aspect | Inheritance | Composition |
---|---|---|
Relationship | Is-a relationship | Has-a relationship |
Flexibility | Less flexible | More flexible |
Reusability | High reusability | High reusability |
Complexity | Can be complex | Generally simpler |
Choose wisely between inheritance and composition based on your design needs. Each approach offers unique strengths.
Virtual Functions And Polymorphism
Virtual functions and polymorphism are key concepts in C++. They help create flexible and reusable code. Understanding these ideas can improve your programming skills. Let’s explore virtual functions and their role in polymorphism.
Understanding Virtual Functions
Virtual functions allow a derived class to override a function in the base class. They ensure the correct function is called for an object. This behavior depends on the type of object, not the type of reference. It makes code more dynamic and adaptable.
To declare a virtual function, use the keyword virtual
in the base class. This signals that the function can be overridden. When you call the function, C++ checks the actual object type. It then executes the appropriate function.
Pure Virtual Functions And Abstract Classes
A pure virtual function is a virtual function without a body. You declare it by assigning 0 in the declaration. This makes the class abstract. Abstract classes cannot be instantiated directly.
Derived classes must implement pure virtual functions. This ensures that the derived class provides specific functionality. Using pure virtual functions encourages a clear design. It defines a common interface for all derived classes.
Runtime Polymorphism
Runtime polymorphism occurs when the program decides which function to call at runtime. This is made possible by virtual functions. It allows for more flexible code and enhances software design.
With runtime polymorphism, you can treat different objects uniformly. You can call the same function on different objects. The correct function will execute based on the object’s type. This leads to cleaner and more maintainable code.
Managing Memory With Oop
Memory management is crucial in C++. It directly impacts program performance. Object-Oriented Programming (OOP) offers several tools for better memory management. This section covers three key areas: dynamic memory allocation, smart pointers, and object copying.
Dynamic Memory Allocation
Dynamic memory allocation allows programs to request memory during runtime. This flexibility is vital for efficient resource use. In C++, developers use the new
and delete
operators for this purpose.
Here is a simple example:
int array = new int[10]; // Allocate memory for an array of 10 integers
// Use the array
delete[] array; // Free the allocated memory
Always remember to free memory. Not doing so can cause memory leaks. This leads to inefficient memory use and crashes.
Smart Pointers
Smart pointers are a safer alternative to raw pointers. They automatically manage memory. This reduces the risk of memory leaks and dangling pointers.
There are three main types of smart pointers:
Type | Description |
---|---|
std::unique_ptr | Owns the memory and cannot be copied. |
std::shared_ptr | Shares ownership of memory with other pointers. |
std::weak_ptr | Does not own memory, helps avoid cycles. |
Using smart pointers simplifies memory management. They ensure memory is released when no longer needed.
Object Copying And The Copy Constructor
Copying objects is common in C++. It often requires special handling. The default copy behavior may not suit all cases. A copy constructor is used to define how objects are copied.
Here’s a simple copy constructor example:
class MyClass {
public:
int value;
MyClass(int val) : value(val) {} // Constructor
MyClass(const MyClass& other) : value(other.value) {} // Copy Constructor
};
In this example, the copy constructor copies the value from another object. Defining a copy constructor can prevent issues like shallow copies. This keeps data consistent and avoids bugs.
Templates In C++ Oop
Templates in C++ allow developers to write flexible and reusable code. They enable functions and classes to operate with any data type. This feature improves code efficiency and reduces duplication. Templates help in creating generic programming structures. Understanding templates is essential for mastering C++ OOP.
Function Templates
Function templates allow you to create functions that can work with any data type. This means you can define a single function that handles different types without rewriting code.
Here’s a simple example:
template
T add(T a, T b) {
return a + b;
}
This function can add integers, floats, or any other type that supports the addition operation.
Key benefits of using function templates:
- Code reusability.
- Easier maintenance.
- Type safety.
Class Templates
Class templates allow you to create classes that can handle any data type. This helps in building data structures that are flexible and reusable.
Here is an example of a class template:
template
class Box {
private:
T item;
public:
Box(T item) : item(item) {}
T getItem() { return item; }
};
In this example, the `Box` class can hold any type of item. You can create boxes for integers, strings, or any other data type.
Benefits of class templates include:
- Enhanced flexibility.
- Reduced code duplication.
- Improved organization.
Template Specialization
Template specialization allows you to define specific behavior for particular data types. This is useful when you need different functionality for specific types.
Here’s how it works:
template
class Calculator {
public:
T add(T a, T b) { return a + b; }
};
// Specialization for type 'std::string'
template <>
class Calculator {
public:
std::string add(std::string a, std::string b) { return a + " and " + b; }
};
In this case, the `Calculator` class has a general definition and a specialized version for strings. This approach provides greater control over how templates behave with different data types.
Advantages of template specialization include:
- Targeted optimization.
- Specific functionality for certain types.
- Better performance.
Best Practices In Oop Design
Object-Oriented Programming (OOP) is a powerful programming paradigm. It helps in organizing code into reusable structures. To create efficient and maintainable code, follow these best practices in OOP design.
Code Reusability
Code reusability is a key benefit of OOP. It saves time and reduces errors. Here are some important points:
- Use inheritance to create new classes from existing ones.
- Utilize interfaces to define common behaviors.
- Implement composition to build complex objects from simpler ones.
By reusing code, you improve productivity. This leads to fewer bugs and easier maintenance.
Maintaining Oop Principles
Stick to the four main principles of OOP:
- Encapsulation: Keep data safe within classes.
- Abstraction: Hide complex details. Show only what is necessary.
- Inheritance: Share common features between classes.
- Polymorphism: Allows methods to do different things based on the object.
Following these principles leads to cleaner and more understandable code.
Design Patterns And Anti-patterns
Design patterns are solutions to common problems in software design. They help in creating flexible and reusable code. Here are some popular design patterns:
Pattern | Description |
---|---|
Singleton | Ensures a class has only one instance. |
Observer | Notifies multiple objects about changes in another object. |
Factory | Creates objects without specifying the exact class. |
Be aware of anti-patterns. They can lead to poor design decisions. Common anti-patterns include:
- Spaghetti Code: Unorganized and hard to follow.
- God Object: A single class that does too much.
- Copy-Paste Programming: Duplicating code instead of reusing it.
Avoid these pitfalls to maintain clean and efficient code.
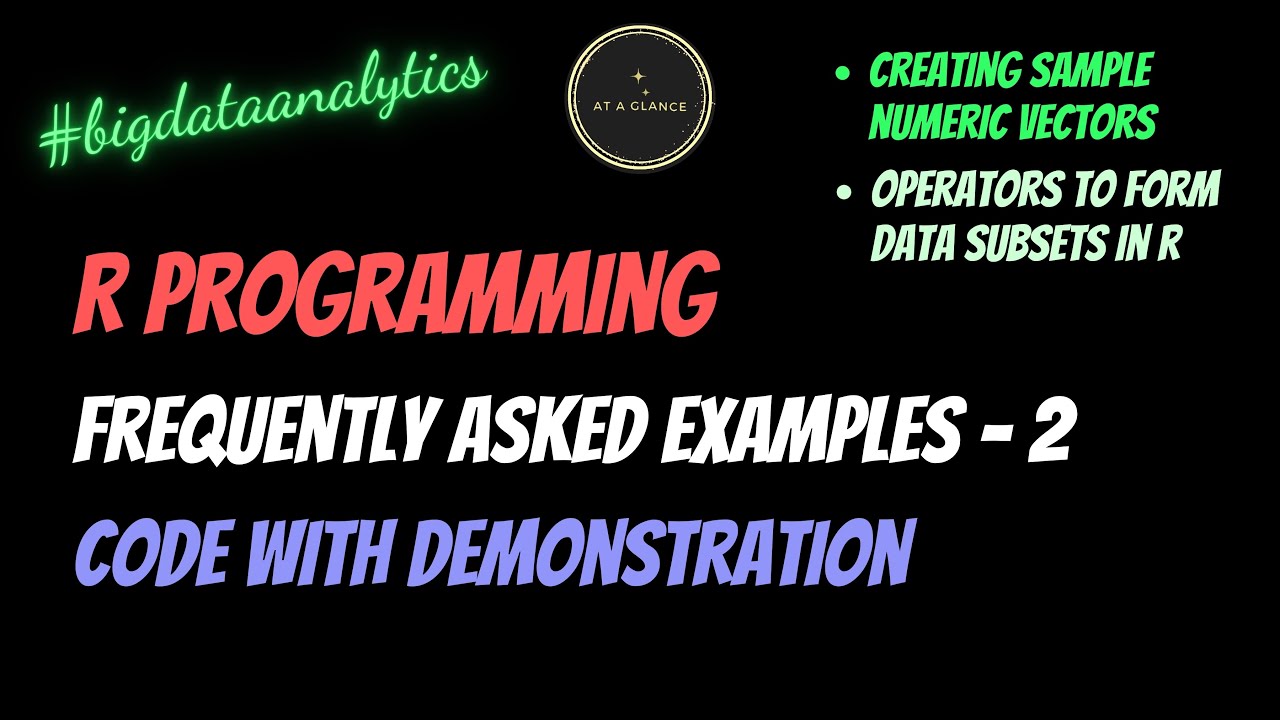
Credit: www.youtube.com
Frequently Asked Questions
What Is Object-oriented Programming In C++?
Object-Oriented Programming (OOP) in C++ is a programming style. It focuses on using objects to design software.
Why Use Oop In C++?
OOP helps organize code better. It makes code easier to understand and maintain.
What Are The Main Features Of Oop?
The main features of OOP are encapsulation, inheritance, and polymorphism. These features help create flexible and reusable code.
Conclusion
Object-Oriented Programming in C++ offers many advantages. It helps organize code better. Classes and objects make it easier to manage complex programs. This approach promotes code reuse and reduces errors. Understanding OOP concepts can improve your programming skills. Start practicing these ideas in your projects.
With time, you will see how useful they are. Embrace the power of C++ and OOP. It can enhance your coding journey greatly. Keep learning and experimenting to grow as a programmer.