Designing a secure web API access is crucial for any website. It protects sensitive data and ensures safe communication between users and services.
APIs, or Application Programming Interfaces, allow different software systems to talk to each other. They are vital for web applications, enabling features like user authentication and data retrieval. However, without proper security measures, APIs can become targets for hackers. This can lead to data breaches and loss of user trust.
Knowing how to design secure API access is essential for any developer or business owner. By following best practices, you can protect your website and its users from potential threats. This guide will walk you through the necessary steps to build secure web API access for your site.
Introduction To Web API Security
Web APIs are essential for modern websites. They allow different systems to communicate. However, they can also be vulnerable to attacks. Understanding web API security is crucial for protecting your data. Secure APIs help keep user information safe. They also build trust with your audience.
The Importance Of Secure Apis
Secure APIs protect sensitive information. They prevent unauthorized access to your system. Weak APIs can lead to data breaches. A data breach can harm your reputation and finances.
Security measures help ensure data integrity. They also maintain the availability of services. Users expect their data to be safe. They will avoid services that do not prioritize security.
Common Threats To Web Apis
APIs face various security threats. One common threat is injection attacks. Attackers can input malicious code to gain access. Another threat is authentication issues. Weak authentication can allow unauthorized users in.
Rate limiting is also a concern. Without it, attackers can overload your API. This can lead to denial of service. It is vital to be aware of these threats. Understanding them helps in designing better security measures.
Principles Of Secure API Design
Designing a secure API is crucial for protecting your website. Following key principles can help you build a strong defense. Focus on limiting access and ensuring data safety. Here are two important principles to consider.
Least Privilege And Minimal Data Exposure
The principle of least privilege means giving users only the access they need. This limits the risk of unauthorized actions. Here’s how to implement it:
- Grant access based on user roles.
- Limit API endpoints to necessary functions.
- Regularly review permissions.
Minimal data exposure reduces the risk of leaks. Only share the data users need. Follow these steps:
- Use data filtering in requests.
- Return only essential fields in responses.
- Scrub sensitive data before sending.
Defense In Depth Approach
A defense in depth approach adds layers of security. Relying on one method is risky. Use multiple strategies to protect your API. Here are common layers:
Layer | Description |
---|---|
Authentication | Verify user identity before granting access. |
Authorization | Ensure users can only access their allowed resources. |
Encryption | Protect data during transfer using SSL/TLS. |
Rate Limiting | Control the number of requests a user can make. |
Implementing these layers reduces vulnerabilities. Strengthen your API against attacks. Always stay updated on security practices.
Authentication Mechanisms
Authentication mechanisms play a crucial role in securing your web API. They verify the identity of users and systems accessing your services. Choosing the right method can protect your data and enhance user trust. This section explores two popular authentication methods: API keys and OAuth tokens, and discusses the implementation of JWT for access control.
Api Keys Vs. Oauth Tokens
API keys and OAuth tokens are common methods for authenticating users. Each method has its own benefits and limitations.
Feature | API Keys | OAuth Tokens |
---|---|---|
Ease of Use | Simple to implement | More complex to set up |
Security Level | Less secure | More secure |
Access Scope | Limited control | Granular control |
Revocation | Requires regeneration | Can revoke easily |
API keys are unique identifiers. They provide basic access control. However, they lack advanced security features.
OAuth tokens allow users to grant access without sharing credentials. They support multiple permissions. OAuth is ideal for applications with sensitive data.
Implementing Jwt For Access Control
JSON Web Tokens (JWT) provide a secure way to transmit information. They are compact, URL-safe tokens that can be verified and trusted. Using JWT improves security in API access.
To implement JWT, follow these steps:
- Generate a token after successful user authentication.
- Sign the token using a secure secret key.
- Send the token to the client for future requests.
- Verify the token on each request to ensure validity.
JWT contains three parts: header, payload, and signature. The header specifies the token type. The payload carries user data. The signature ensures the token’s integrity.
Benefits of using JWT:
- Stateless authentication
- Reduced server load
- Easy to use across different platforms
Implementing strong authentication mechanisms is essential. Choose the right method based on your needs. Protect your API and your users effectively.
Authorization Strategies
Authorization strategies are vital for securing your web API. They define who can access what. Proper strategies help protect sensitive data and resources. Two common methods are Role-Based Access Control and Attribute-Based Access Control. Each has unique benefits and uses.
Role-based Access Control (rbac)
Role-Based Access Control (RBAC) assigns permissions based on user roles. Roles group users with similar access needs. For example, an admin role may have full access. A guest role might only have limited access. This method simplifies management. It is easy to add or remove roles as needed.
RBAC works well for organizations with clear hierarchies. It reduces the chance of human error. By defining roles, you streamline access control. This method is straightforward. It helps ensure that users only see what they need.
Attribute-based Access Control (abac)
Attribute-Based Access Control (ABAC) uses user attributes for permissions. These attributes can be user location, time, or device type. ABAC allows more flexible access control. It adapts to different situations and contexts.
This method provides a fine-grained approach. You can set detailed rules. For instance, a user may access data only during working hours. Or, allow access only from specific devices. ABAC is powerful for complex systems. It meets diverse security needs.
Securing Data In Transit
Securing data in transit is vital for protecting sensitive information. Data travels over the internet and can be intercepted. Using proper security measures reduces this risk. The right protocols keep your data safe from unauthorized access.
The Role Of Ssl/tls
SSL (Secure Sockets Layer) and TLS (Transport Layer Security) are protocols. They encrypt data sent between a user’s browser and your server. This encryption prevents third parties from reading the data.
- SSL/TLS creates a secure channel.
- It authenticates the server’s identity.
- It ensures data is encrypted before transmission.
Implementing SSL/TLS is essential for:
- Protecting user information like passwords and credit card numbers.
- Building trust with users through secure connections.
- Improving SEO rankings, as search engines favor secure sites.
Ensuring Data Integrity With Https
HTTPS (HyperText Transfer Protocol Secure) is the secure version of HTTP. It uses SSL/TLS to encrypt data. This ensures that the data sent and received is not altered during transmission.
Feature | HTTP | HTTPS |
---|---|---|
Encryption | No | Yes |
Data Integrity | No | Yes |
Authentication | No | Yes |
Switching to HTTPS offers several benefits:
- Protects against man-in-the-middle attacks.
- Ensures data is not tampered with.
- Enhances user confidence in your website.
Securing data in transit is not just a choice. It is a necessity. Using SSL/TLS and HTTPS protects your users. It also protects your business from data breaches.
Credit: idratherbewriting.com
Validating And Sanitizing Input
Validating and sanitizing input is crucial for web API security. This process ensures that user data is checked and cleaned before processing. It helps prevent attackers from exploiting vulnerabilities. Proper input validation can protect your website from various threats.
Preventing Injection Attacks
Injection attacks are common threats. They occur when an attacker sends malicious data. This can disrupt your web application. Here are some types of injection attacks:
- SQL Injection: The attacker inserts SQL code to manipulate your database.
- Command Injection: The attacker executes system commands on your server.
- Cross-Site Scripting (XSS): The attacker injects scripts into web pages viewed by users.
To prevent these attacks:
- Always use prepared statements for database queries.
- Escape user input before using it in commands.
- Implement a web application firewall (WAF).
Input Validation Best Practices
Effective input validation keeps your data safe. Follow these best practices:
Practice | Description |
---|---|
Whitelist Input | Accept only specific, safe input values. |
Limit Input Length | Restrict the number of characters accepted. |
Use Type Checking | Verify that the input is of the correct data type. |
Regular Expressions | Use regex to define valid input patterns. |
Sanitize input by removing or encoding dangerous characters. This reduces the risk of attacks. Always validate both client-side and server-side. Client-side checks improve user experience. Server-side checks provide security against bypassing.
Error Handling And Logging
Effective error handling and logging are vital for a secure web API. They help identify issues, track user actions, and protect sensitive data. A well-designed system can prevent attackers from gaining valuable information. This section covers key aspects of error handling and logging.
Avoiding Information Leakage
Information leakage occurs when error messages reveal too much detail. Attackers can exploit this information. Follow these guidelines to prevent it:
- Generic Error Messages: Use non-specific messages for users. Example: “An error occurred. Please try again.”
- Do Not Include Stack Traces: Avoid showing technical details. They can expose vulnerabilities.
- Log Detailed Errors Securely: Store detailed errors in logs. Ensure logs are secure and accessible only to authorized personnel.
By avoiding information leakage, you reduce risks and protect your web API.
Maintaining Audit Trails
Audit trails track user activities in your API. They help in monitoring and diagnosing issues. Here are key points to consider:
- Log All Requests: Record each API request. Include timestamps, user IDs, and actions.
- Store Logs Securely: Protect log files. Use encryption and limit access to trusted users.
- Review Logs Regularly: Analyze logs for unusual activities. Look for failed login attempts and unauthorized access.
Implementing robust audit trails enhances security. It provides a clear history of interactions with your API.
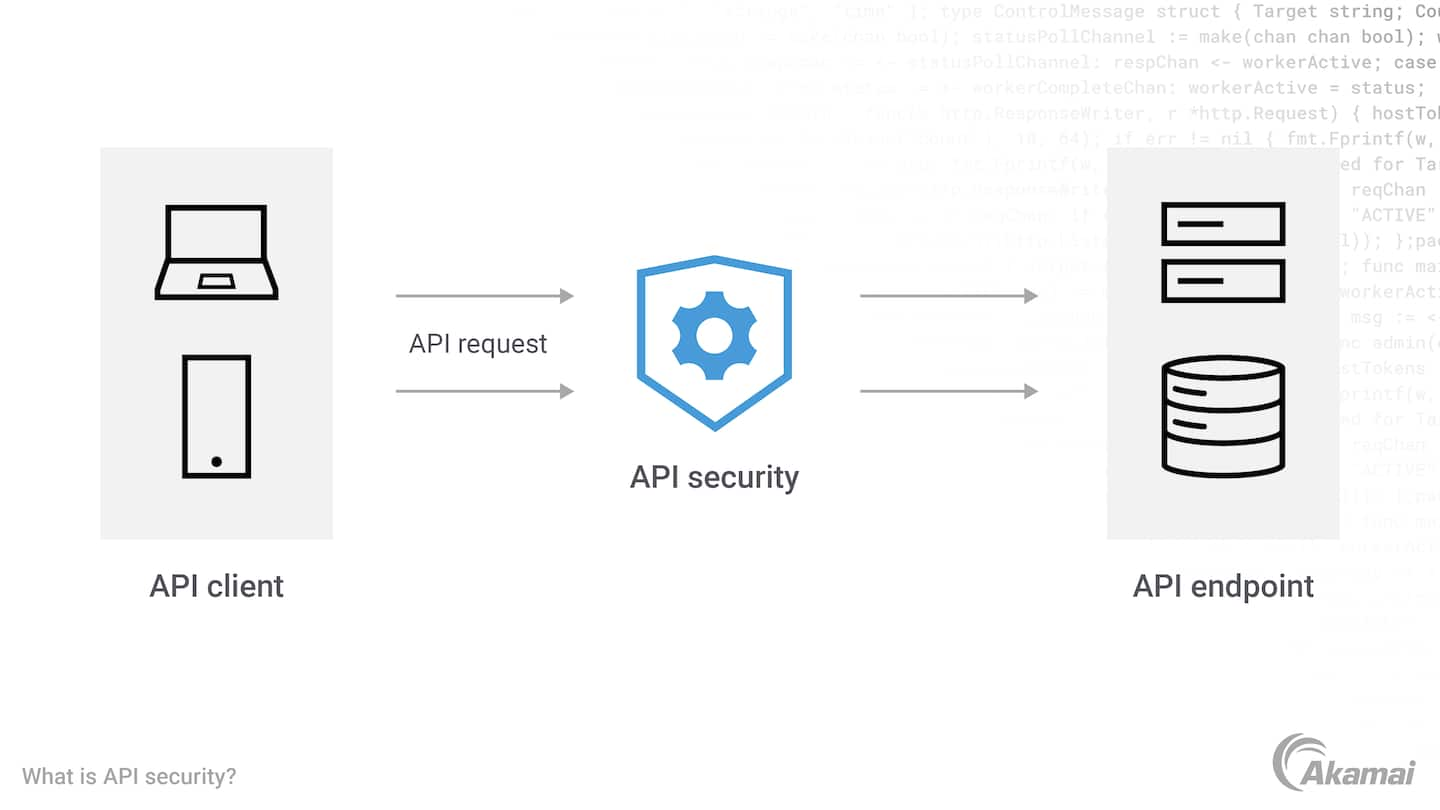
Credit: www.akamai.com
Rate Limiting And Throttling
Rate limiting and throttling are key parts of API security. They help control how many requests users can make. This reduces the risk of abuse and keeps your API running smoothly. A well-designed rate-limiting system can protect your website from many attacks.
Protecting Against Denial Of Service
Denial of Service (DoS) attacks aim to overwhelm your server. Attackers send too many requests, causing your service to crash. Rate limiting helps stop this by restricting the number of requests from a single user.
By setting a limit on requests, you ensure fair use. This keeps your website responsive for all users. Implementing a robust rate-limiting strategy is crucial for maintaining uptime.
Configuring API Rate Limits
Configuring API rate limits is straightforward. Start by identifying your traffic patterns. Then, set limits based on user roles. For example, regular users may have lower limits than premium users.
Use a clear policy for your limits. Inform users about their allowed requests. This transparency builds trust and helps prevent frustration.
Monitor your API usage regularly. Adjust limits as needed based on real-time data. This keeps your API secure and efficient.
API Security Tools And Testing
Securing your API is vital for your website’s safety. Many tools help with this task. Testing your API for vulnerabilities keeps your data safe. Using the right tools can make a big difference. Regular testing ensures that your API remains secure.
Automated Security Scanning
Automated security scanning is a key part of API security. These tools check your API for common vulnerabilities. They run tests quickly and efficiently. This saves time and helps find issues early.
Popular tools include OWASP ZAP and Burp Suite. These tools scan for weak points. They also provide reports on vulnerabilities. Regular scans help maintain API security over time.
Penetration Testing For Apis
Penetration testing is another important step. This involves simulating attacks on your API. Skilled testers look for weaknesses and possible exploits. They try to breach your API, revealing security gaps.
Using penetration testing tools like Postman can enhance security. These tools allow for detailed testing of API endpoints. Understanding how attackers might exploit your API is crucial. It helps you strengthen your defenses.
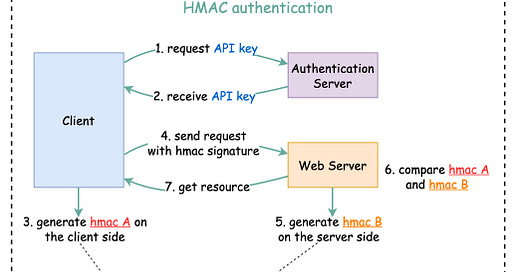
Credit: blog.bytebytego.com
Maintaining Api Security
Maintaining API security is crucial for protecting your website. It helps guard sensitive data and prevents unauthorized access. Here are two essential practices to ensure your API remains secure.
Regular Security Audits
Conducting regular security audits is vital. This process helps identify weaknesses in your API. Here are steps to perform effective audits:
- Review your API documentation.
- Check access controls and permissions.
- Test for vulnerabilities using automated tools.
- Assess third-party libraries for security flaws.
Schedule audits at least once a year. More frequent checks are better, especially after major changes. Document findings and address issues promptly.
Staying Updated With Security Patches
Keeping your software updated is essential. Security patches fix known vulnerabilities. Follow these tips:
- Subscribe to security newsletters.
- Use version control for your API.
- Implement a patch management system.
- Test patches in a staging environment.
Apply updates quickly. Outdated software can become an easy target for attackers. Regular updates help maintain a strong security posture.
Frequently Asked Questions
How Can I Secure My Web API Access?
Secure your web API by using authentication methods like OAuth or API keys. Limit access to trusted users only.
What Are Common Web Api Security Risks?
Common risks include unauthorized access, data leaks, and denial-of-service attacks. Regularly update your security measures to protect against these threats.
Why Is HTTPS Important For Web Apis?
HTTPS encrypts data during transmission. This prevents eavesdropping and protects sensitive information from being intercepted.
Conclusion
A secure web API is vital for your website. It protects your data and your users. Use strong authentication methods. Always validate input and limit access. Regularly test for vulnerabilities. Monitor usage to spot any unusual activity. Keep your software updated.
These steps help you build a safer web environment. Remember, security is an ongoing process. Stay informed about new threats. By prioritizing security, you can create trust with your users. A secure API leads to a better online experience for everyone.