Learning Python can seem daunting at first. Yet, with a clear plan, anyone can do it.
This blog post presents a 30-day master plan that breaks down the learning process into manageable steps. Python is a popular programming language, known for its simplicity and versatility. Many people use it for web development, data analysis, and automation.
This guide will help you learn Python effectively over 30 days. Each day focuses on specific topics and skills. You will build a strong foundation and develop practical skills. Whether you are a beginner or want to refresh your knowledge, this plan is for you. Follow along, and you will see progress in just one month. Let’s dive into your journey of learning Python!
Introduction To The 30-day Python Challenge
Welcome to the 30-Day Python Challenge! This plan helps you learn Python step by step. It is perfect for beginners. Each day, you will tackle a new topic. By the end, you will have a solid understanding of Python.
Why Python Is A Great Choice For Beginners
Python is one of the easiest programming languages to learn. Here are some reasons why:
- Simple Syntax: Python uses clear and readable code.
- Large Community: Many people use Python. You can find help easily.
- Versatile: Python works for web, data, and AI projects.
- Great Libraries: Python has many libraries to simplify tasks.
These features make Python a great choice for beginners. You can start coding quickly and see results fast.
Goals And Expectations For The Next 30 Days
Here’s what you can expect during this challenge:
Week | Topics | Goals |
---|---|---|
Week 1 | Basics of Python | Understand syntax and basic commands. |
Week 2 | Data Types and Variables | Learn about strings, lists, and dictionaries. |
Week 3 | Control Flow | Use loops and conditionals in Python. |
Week 4 | Functions and Modules | Create reusable code and organize projects. |
Set realistic goals for yourself. Aim to spend at least 30 minutes each day coding. Practice is key to success.
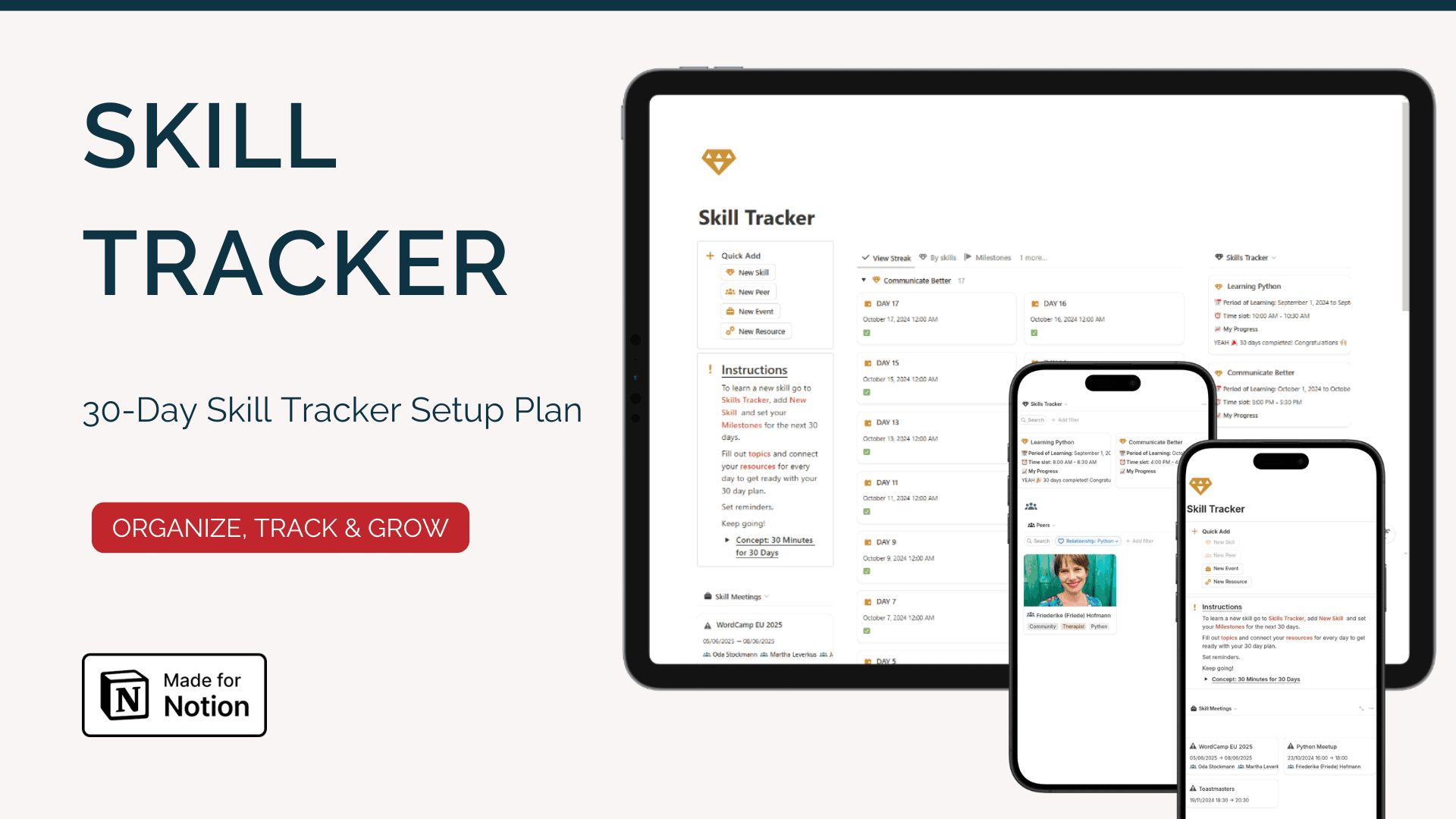
Credit: www.notion.com
Getting Started With Python
Starting your journey with Python is exciting. Python is a popular programming language. It is simple and easy to learn. This section will help you set up and understand Python basics.
Setting Up Your Development Environment
To begin, you need to set up your development environment. This is where you will write and run your Python code. First, download Python from the official website. Choose the version suitable for your operating system.
Next, install a code editor. Popular choices are VS Code, PyCharm, or even IDLE, which comes with Python. These editors help you write code easily.
After installation, open your code editor. Create a new file and save it with a .py extension. This tells your system it’s a Python file. You are now ready to write your first Python program.
Understanding Python Syntax Basics
Python syntax is straightforward. You will find it easy to read and write. Start with printing text. Use the print() function for this. For example, type print("Hello, World!")
.
Indentation matters in Python. It shows code blocks. Always use four spaces or a tab for indentation. This keeps your code organized.
Learn about variables next. Variables store data. You can create one by typing a name followed by an equals sign. For example, name = "Alice"
assigns “Alice” to the variable name.
Explore data types too. Python supports strings, integers, lists, and more. Each type has its uses. Understanding these basics helps you write better code.
Week 1: Python Fundamentals
Welcome to the first week of your Python journey. This week focuses on the basics. You will learn key concepts that form the foundation of Python programming. Understanding these concepts is crucial. They will help you as you progress.
Variables, Data Types, And Operators
Variables are like containers for data. You can store different types of information in them. Python has several data types. These include integers, floats, strings, and booleans.
Integers are whole numbers. Floats are numbers with decimals. Strings are text, while booleans represent True or False.
Operators allow you to perform calculations and comparisons. You can add, subtract, multiply, or divide numbers. You can also compare values using operators like equal to, less than, or greater than.
Control Flow: If Statements And Loops
Control flow directs the order of execution in your code. If statements help make decisions. They allow your program to respond based on conditions.
For example, you can check if a number is positive or negative. Based on the result, your program can take different actions.
Loops let you repeat tasks. The two main types are for loops and while loops. For loops iterate over a sequence. While loops continue until a condition is false.
These concepts are essential for writing effective code. Mastering them will make programming easier.
Credit: github.com
Week 1 Projects: Simple Python Programs
In the first week, focus on creating simple Python programs. These projects help you practice basic coding skills. You will learn to write code and solve problems. Start with two fun projects: a calculator and a guessing game. Both will build your confidence in Python.
Building A Calculator
Creating a calculator is a great first project. It helps you understand basic operations. You will use addition, subtraction, multiplication, and division.
Here’s a simple way to build your calculator:
- Define functions for each operation.
- Ask the user for numbers and the operation.
- Perform the operation and display the result.
Here is a sample code for your calculator:
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x y
def divide(x, y):
return x / y if y != 0 else "Cannot divide by zero"
print("Select operation:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
choice = input("Enter choice (1/2/3/4): ")
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
if choice == '1':
print("Result:", add(num1, num2))
elif choice == '2':
print("Result:", subtract(num1, num2))
elif choice == '3':
print("Result:", multiply(num1, num2))
elif choice == '4':
print("Result:", divide(num1, num2))
else:
print("Invalid input")
Creating A Basic Guessing Game
A guessing game is another fun project. It teaches you about loops and conditions. The player guesses a number within a range. The program gives hints if the guess is too high or too low.
Follow these steps to create your game:
- Import the random module.
- Set a number range for guessing.
- Ask the player for a guess.
- Check if the guess is correct.
Here’s a basic code for your guessing game:
import random
number_to_guess = random.randint(1, 100)
guess = 0
while guess != number_to_guess:
guess = int(input("Guess a number between 1 and 100: "))
if guess < number_to_guess:
print("Too low!")
elif guess > number_to_guess:
print("Too high!")
else:
print("Congratulations! You guessed it right.")
These projects are simple but very effective. They help you practice your skills. Enjoy coding!
Week 2: Diving Deeper Into Python
In Week 2, we focus on important Python concepts. You will learn about functions and modules. Understanding these topics will help you write better code. You will also explore file handling and exception management.
Functions And Modules
Functions are blocks of code that perform a specific task. They help organize your code and make it reusable. You can define a function using the def
keyword. After defining a function, you can call it anytime.
Modules are files that contain Python code. They can include functions, classes, or variables. You can import modules to use their features in your project. This saves time and effort. The built-in math
module is a good example.
Creating your modules is easy. Just save your functions in a .py file. Use them import
to bring them into your main program. This practice makes your code cleaner and more organized.
Working With Files And Exception Handling
Python allows you to work with files easily. You can read from and write to files. Use the open()
function to access files. Specify the mode: read, write, or append.
Reading a file gives you access to its content. Writing to a file saves your data. Always close the file after using it. This prevents data loss and resource leaks.
Exception handling is crucial in programming. It helps manage errors gracefully. Use try
and except
blocks to catch exceptions. This way, your program won’t crash unexpectedly.
Practice these concepts during Week 2. Understanding functions, modules, file handling, and exceptions is vital. These skills will boost your Python programming journey.
Week 2 Projects: Intermediate Python Projects
In Week 2, focus on building your skills with intermediate Python projects. These projects will help you apply what you learned in Week 1. You will create useful applications and dive deeper into Python’s capabilities. This week includes two main projects: a To-Do list application and a data analysis project. Both will enhance your coding skills and confidence.
Developing A To-do List Application
A To-Do list application is a great project for beginners. It teaches you about functions, lists, and user input. Start by creating a simple command-line version. Users should be able to add, remove, and view tasks.
Use lists to store tasks. Each task can be a string in the list. Create functions for adding and removing tasks. Then, build a menu to show options. Encourage users to interact with the program. This will make the project more engaging.
Once you finish the command-line version, consider adding features. You can add due dates or priorities to tasks. Think about saving tasks to a file. This way, users can keep their lists even after closing the app.
Data Analysis With Python
Data analysis is an exciting area to explore. It allows you to turn data into insights. You can use libraries like Pandas and Matplotlib. Start with a simple dataset. Look for open datasets online.
Load the dataset into Python using Pandas. Learn to manipulate data with basic functions. You can filter, sort, and group data. Try to find interesting trends or patterns.
Visualize your findings with Matplotlib. Create charts to display your data. This will help you understand the information better. Aim to answer a specific question with your analysis.
These projects will boost your confidence. They show how Python can solve real problems. Keep practicing and experimenting with new ideas.
Week 3: Object-oriented Programming
Welcome to Week 3 of your Python learning journey. This week, you will dive into Object-Oriented Programming (OOP). OOP is a key concept in Python. It helps you organize your code better. You will learn about classes and objects. You will also explore inheritance and polymorphism. These concepts will help you write cleaner and more efficient code.
Classes And Objects
Classes are blueprints for creating objects. They define properties and behaviors. An object is an instance of a class. It can hold data and perform actions.
To create a class in Python, use the class
keyword. Define its properties inside the class. Use methods to define its behavior. Here is a simple example:
class Dog:
def __init__(self, name):
self.name = name
def bark(self):
return "Woof!"
In this example, Dog
is a class. It has a property called name
. The method bark
allows the dog to bark. Create an object of the class to use it:
my_dog = Dog("Buddy")
print(my_dog.bark()) # Output: Woof!
Inheritance And Polymorphism
Inheritance allows one class to inherit properties from another. This helps avoid code duplication. For example, you can create a class Animal
and have Dog
inherit from it:
class Animal:
def speak(self):
return "Animal speaks"
class Dog(Animal):
def speak(self):
return "Woof!"
In this case, Dog
inherits from Animal
. It also overrides the speak
method. This is an example of polymorphism.
Polymorphism allows methods to do different things based on the object. Different classes can have methods with the same name. Each class can implement it in its own way.
Understanding these concepts is important. They form the foundation of OOP in Python. Practice creating classes and using inheritance. This will make your code more flexible and easier to manage.
Credit: www.instagram.com
Week 3 Projects: Oop Concepts In Action
In Week 3, you will focus on projects that use Object-Oriented Programming (OOP). This will help you understand how to create and use classes and objects. You will work on two fun projects: an Inventory System and a Text-Based Adventure Game. Both projects will strengthen your coding skills.
Designing A Simple Inventory System
The first project is a simple Inventory System. This system will help track items in a store or warehouse. You will learn how to create classes and methods. Below are the main features you will implement:
- Add items to the inventory.
- Remove items from the inventory.
- Display all items in the inventory.
Here is a sample code snippet to help you get started:
class Item:
def __init__(self, name, quantity):
self.name = name
self.quantity = quantity
class Inventory:
def __init__(self):
self.items = []
def add_item(self, item):
self.items.append(item)
def remove_item(self, item_name):
self.items = [item for item in self.items if item.name != item_name]
def display_inventory(self):
for item in self.items:
print(f'{item.name}: {item.quantity}')
This code shows how to create an Item class and an Inventory class. You can expand this system with more features later.
Building A Text-based Adventure Game
The second project is a Text-Based Adventure Game. This game allows players to explore a story through text. You will create rooms, items, and characters. Here are the key elements:
- Create different rooms for players to explore.
- Add items that players can collect.
- Include characters that players can interact with.
Below is a simple example of how to set up the game:
class Room:
def __init__(self, name, description):
self.name = name
self.description = description
self.items = []
class Game:
def __init__(self):
self.rooms = []
self.current_room = None
def add_room(self, room):
self.rooms.append(room)
def set_starting_room(self, room):
self.current_room = room
def play(self):
while True:
print(self.current_room.description)
# Add game logic here
This code shows a basic structure for your game. You can build on this by adding more features. Think about quests, puzzles, and challenges.
Week 4: Advanced Python Topics
In Week 4, the focus shifts to advanced Python topics. This week covers web development with Flask and data visualization. Both are essential skills for Python developers. Let’s dive into these areas to expand your knowledge.
Exploring Web Development With Flask
Flask is a popular web framework for Python. It is lightweight and easy to use. This makes it ideal for beginners.
- Lightweight: Flask has fewer components than other frameworks.
- Flexible: You can build applications your way.
- Easy to learn: Simple syntax helps you get started quickly.
Here are some key concepts to learn:
- Setting up Flask: Install Flask using pip.
- Creating a basic app: Learn how to create a simple web app.
- Routing: Understand how to handle different URLs.
- Templates: Use Jinja2 to create dynamic web pages.
Example code to create a basic Flask app:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return "Hello, Flask!"
if __name__ == '__main__':
app.run(debug=True)
Start building your first web app with Flask. Practice the concepts learned. This will enhance your Python skills.
Introduction To Data Visualization
Data visualization helps to present data. Python has several libraries for this task. Popular libraries include Matplotlib and Seaborn.
Library | Description |
---|---|
Matplotlib | Basic plotting library for creating graphs. |
Seaborn | Built on Matplotlib, it creates more attractive graphs. |
Pandas | Great for data manipulation and basic plotting. |
Here are steps to create a simple plot:
- Install Matplotlib: Use pip to install.
- Import the library: Start your script with import.
- Prepare data: Create lists or arrays for your data.
- Plot the data: Use the plot function to create a graph.
Sample code to create a basic line plot:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4]
y = [10, 20, 25, 30]
plt.plot(x, y)
plt.title("Simple Line Plot")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.show()
Start visualizing your data. Use the libraries to create different charts. This will help you understand data better.
Final Week: Capstone Project And Beyond
The final week of your 30-day Python learning plan is here. This week focuses on your Capstone Project. You will apply everything you learned. This is an exciting chance to show your skills. It is also an opportunity to explore new ideas.
Planning And Executing A Capstone Project
Start by picking a project that interests you. It should be simple but challenging. Here are some steps to help you:
- Choose a Topic: Pick something you love.
- Set Goals: Decide what you want to achieve.
- Break It Down: Divide the project into small tasks.
- Research: Look for resources and examples.
- Write Code: Start coding step by step.
- Test: Check your code for errors.
- Document: Explain your code and decisions.
Use a simple table to track your progress:
Task | Status | Notes |
---|---|---|
Choose a Topic | Completed | Selected a weather app |
Set Goals | In Progress | Define main features |
Research | Not Started | Look for APIs |
Keep your project simple. Focus on learning. You can always expand it later.
Next Steps: Continuing Your Python Journey
After finishing your Capstone Project, think about what comes next. Here are some ideas:
- Explore Advanced Topics: Learn about web development or data science.
- Contribute to Open Source: Join projects on platforms like GitHub.
- Join a Community: Engage with other Python learners.
- Practice Regularly: Solve problems on coding websites.
- Build More Projects: Create apps or tools that interest you.
Keep your skills sharp. Challenge yourself with new projects. Stay curious and keep learning.
Frequently Asked Questions
How Long Does It Take To Learn Python?
Learning Python can take about a month with daily practice. A solid plan helps you stay focused and motivated.
What Are The Best Resources For Learning Python?
Great resources include online courses, tutorials, and books. Websites like Codecademy and Coursera offer structured lessons.
Can I Learn Python Without Prior Coding Experience?
Yes, you can learn Python without any coding background. Python is beginner-friendly and easy to understand.
Conclusion
Learning Python in 30 days is possible. Stick to the plan. Each day brings new skills. Practice makes a big difference. Use resources like books and online tutorials. Join a community for support. Ask questions when you’re stuck. Celebrate small wins to stay motivated.
Keep coding and learning. Your journey in Python can lead to many opportunities. Start today, and enjoy the process.