Microservices work together to build complex applications. Each microservice has its function and can operate independently.
Understanding how microservices collaborate is essential for modern software development. These small, self-contained services communicate and share data to create a cohesive system. They can do this through APIs, message queues, and service discovery tools. This approach allows teams to develop, test, and deploy services quickly and efficiently.
As organizations adopt microservices, knowing how they interact becomes crucial. It helps in designing systems that are flexible and scalable. This blog post will explore the various ways microservices work together, ensuring you have a clear picture of their interactions and collaboration methods.
Microservices Architecture: The Basics
Microservices architecture is a way to design software. It breaks applications into small, independent services. Each service performs a specific function. They work together to form a complete application. This structure allows teams to work on different parts at the same time. It makes development faster and easier.
Core Principles
Microservices follow some core principles:
- Independence: Each service can run on its own.
- Small Size: Services are small and focused on one task.
- Loose Coupling: Services interact but do not rely heavily on each other.
- Technology Agnostic: Teams can use different technologies for each service.
- Scalable: Services can be scaled independently based on demand.
Benefits Of Scalability And Flexibility
Microservices offer several benefits for scalability and flexibility:
Benefit | Description |
---|---|
Easy Scaling | Scale services based on traffic needs. |
Faster Deployments | Deploy updates to one service without affecting others. |
Improved Fault Isolation | If one service fails, others can continue to work. |
Team Autonomy | Teams can work on services without waiting on others. |
Technology Diversity | Use different technologies best suited for each service. |
Microservices architecture enhances both scalability and flexibility. Teams can adapt quickly to changing needs. This approach improves the overall efficiency of software development.
Microservices Communication: Synchronous Vs Asynchronous
Microservices communication is vital for seamless interaction between services. Each microservice can operate independently. They must still share data and functionality. Two main types of communication exist: synchronous and asynchronous. Understanding these methods helps in building efficient microservices.
API Gateways
An API Gateway acts as a bridge. It connects clients to microservices. This gateway handles requests and responses. It can provide a unified entry point for users.
- Request Routing: Directs requests to the appropriate microservice.
- Authentication: Ensures secure access to services.
- Load Balancing: Distributes traffic evenly across instances.
- Response Aggregation: Combines responses from multiple services.
API Gateways can support both synchronous and asynchronous communication. For synchronous calls, they wait for responses. For asynchronous calls, they send requests and continue processing.
Event-driven Approaches
Event-driven architectures enable services to communicate without direct connections. Services publish events to a message broker. Other services can subscribe to these events. This method enhances decoupling between services.
- Loose Coupling: Services operate independently.
- Scalability: Easily add more services without disruption.
- Resilience: If one service fails, others continue to work.
In this approach, services do not wait for responses. They react to events as they occur. This leads to faster processing and improved user experiences.
Both API Gateways and event-driven approaches play critical roles. They provide flexibility in how microservices communicate. Choosing the right method depends on specific needs.
Service Discovery In A Microservices Ecosystem
Service discovery plays a key role in a microservices ecosystem. It helps services find and communicate with each other. As services scale and change, a robust discovery mechanism is essential. Two common methods for service discovery are client-side discovery and server-side discovery. Each method has its unique approach to managing service interactions.
Client-side Discovery
In client-side discovery, the client takes charge of finding services. The client knows where to look for services and how to connect. Here is how it works:
- The client queries a service registry.
- The service registry contains a list of available services.
- The client receives the service instance details.
- The client connects directly to the service instance.
This method places the responsibility on the client. It requires more logic in the client code. Benefits of client-side discovery include:
- Reduced load on servers.
- Direct communication between client and service.
Server-side Discovery
In server-side discovery, the client does not find services directly. Instead, it sends requests to a load balancer or API gateway. Here’s how server-side discovery operates:
- The client requests the load balancer.
- The load balancer queries the service registry.
- The load balancer forwards the request to an available service instance.
Server-side discovery simplifies client code. It centralizes the discovery logic in the server. This method has advantages such as:
- Less complexity in client applications.
- Easier management of service instances.
Discovery Method | Pros | Cons |
---|---|---|
Client-Side Discovery | Reduced server load, direct connections | The more complex client logic |
Server-Side Discovery | Simplified client code, centralized logic | Potential bottleneck at load balancer |
Choosing between client-side and server-side discovery depends on your architecture. Each method has its strengths and weaknesses. Understanding both helps in making informed decisions.
Inter-service Communication Protocols
Microservices need to communicate with each other to function well. This communication happens through various protocols. These protocols define how services send and receive data. Understanding these protocols helps in building efficient systems.
Rest Apis
REST (Representational State Transfer) APIs are popular in microservices. They use standard HTTP methods like GET, POST, PUT, and DELETE. REST is simple and easy to use. Here are some key points about REST APIs:
- Stateless: Each request from a client to a server must contain all the information needed to understand it.
- Resource-based: Everything is treated as a resource. Each resource has a unique URL.
- JSON format: Most REST APIs use JSON for data exchange, making it lightweight.
REST APIs work well for web services. They allow different services to interact smoothly.
Grpc
gRPC is another communication protocol. It is based on HTTP/2 and uses Protocol Buffers for data serialization. gRPC offers some advantages:
- Fast: It supports streaming and reduces latency.
- Strongly typed: It enforces strict types, reducing errors.
- Bi-directional streaming: Clients and servers can send messages simultaneously.
gRPC is ideal for connecting services in real-time applications.
Message Brokers
Message brokers help microservices communicate asynchronously. They store messages and deliver them to the right service. Common message brokers include RabbitMQ and Apache Kafka. Here’s how they work:
Feature | RabbitMQ | Apache Kafka |
---|---|---|
Message Delivery | Queue-based | Topic-based |
Use Case | Transactional systems | Real-time data streaming |
Performance | Moderate | High |
Message brokers improve reliability and scalability. They allow services to work independently. This flexibility is crucial in a microservices architecture.
Data Sharing And Management Strategies
Data sharing is crucial in microservices. Each service needs to communicate with others. Effective management strategies help maintain data integrity and consistency. Let’s explore three main strategies.
Database Per Service
Each microservice often has its database. This approach keeps services independent. Changes in one database won’t affect others. It allows teams to choose the best database for their needs. This strategy enhances scalability. Services can evolve without disrupting the entire system.
Api Composition
API composition is another way to share data. Services communicate through APIs. An API gathers data from various services. It returns a single response to the user. This method simplifies data access. It also reduces the complexity of interactions. Developers can focus on individual services without worrying about others.
Command Query Responsibility Segregation (CQRS)
CQRS separates reading and writing data. One model handles commands, while another manages queries. This distinction improves performance. Each model can be optimized for its purpose. It allows for more efficient data handling. Services can scale independently, based on their needs.
Credit: twitter.com
Fault Tolerance And Resilience Patterns
Microservices often work together. They must handle failures well. This is where fault tolerance and resilience patterns come in. These patterns help services keep running smoothly, even if one service fails. They ensure that the overall system remains stable. Understanding these patterns is crucial for building robust applications.
Circuit Breaker Pattern
The Circuit Breaker Pattern prevents a service from trying to execute an operation that is likely to fail. It acts like an electrical circuit breaker. Here’s how it works:
- Closed State: The service operates normally.
- Open State: If errors exceed a threshold, the circuit opens. The service stops trying to connect.
- Half-Open State: After a period, it allows a limited number of attempts. If successful, it goes back to closed. If not, it returns to open.
This pattern protects services from cascading failures. It helps maintain the overall health of the system.
Bulkhead Pattern
The Bulkhead Pattern divides a system into isolated components. Think of it like compartments in a ship. If one compartment leaks, others stay dry. Here’s how it benefits microservices:
- Isolation: Each service operates independently.
- Resource Allocation: Limits the resources each service can use.
- Failure Containment: Prevents one service’s failure from affecting others.
This pattern increases the system’s resilience. It allows services to fail without bringing down the entire system.
Pattern | Description | Benefits |
---|---|---|
Circuit Breaker | Stops call to a failing service. | Prevents cascading failures. |
Bulkhead | Isolates services into compartments. | Contains failures and protects other services. |
Monitoring And Observability In Microservices
Monitoring and observability are crucial in microservices. They help track performance and health. Understanding how services work together is key. Effective monitoring identifies issues early. Observability provides insights into system behavior.
Logging
Logging captures important events in microservices. It helps in tracking what happens during execution. Good logging practices improve troubleshooting. Here are some important points:
- Log important events and errors.
- Use a structured format for logs.
- Include timestamps and service names.
- Centralize logs for easy access.
Centralized logging tools like ELK Stack or Splunk are helpful. They gather logs from all services. This way, you can see the complete picture.
Distributed Tracing
Distributed tracing allows tracking requests across services. It shows how requests flow through microservices. This helps identify bottlenecks and latency issues. Key benefits include:
- Visualize request paths.
- Identify slow services.
- Understand service dependencies.
Tools like Jaeger and Zipkin help with distributed tracing. They provide clear insights into how services interact. This makes it easier to find and fix problems.
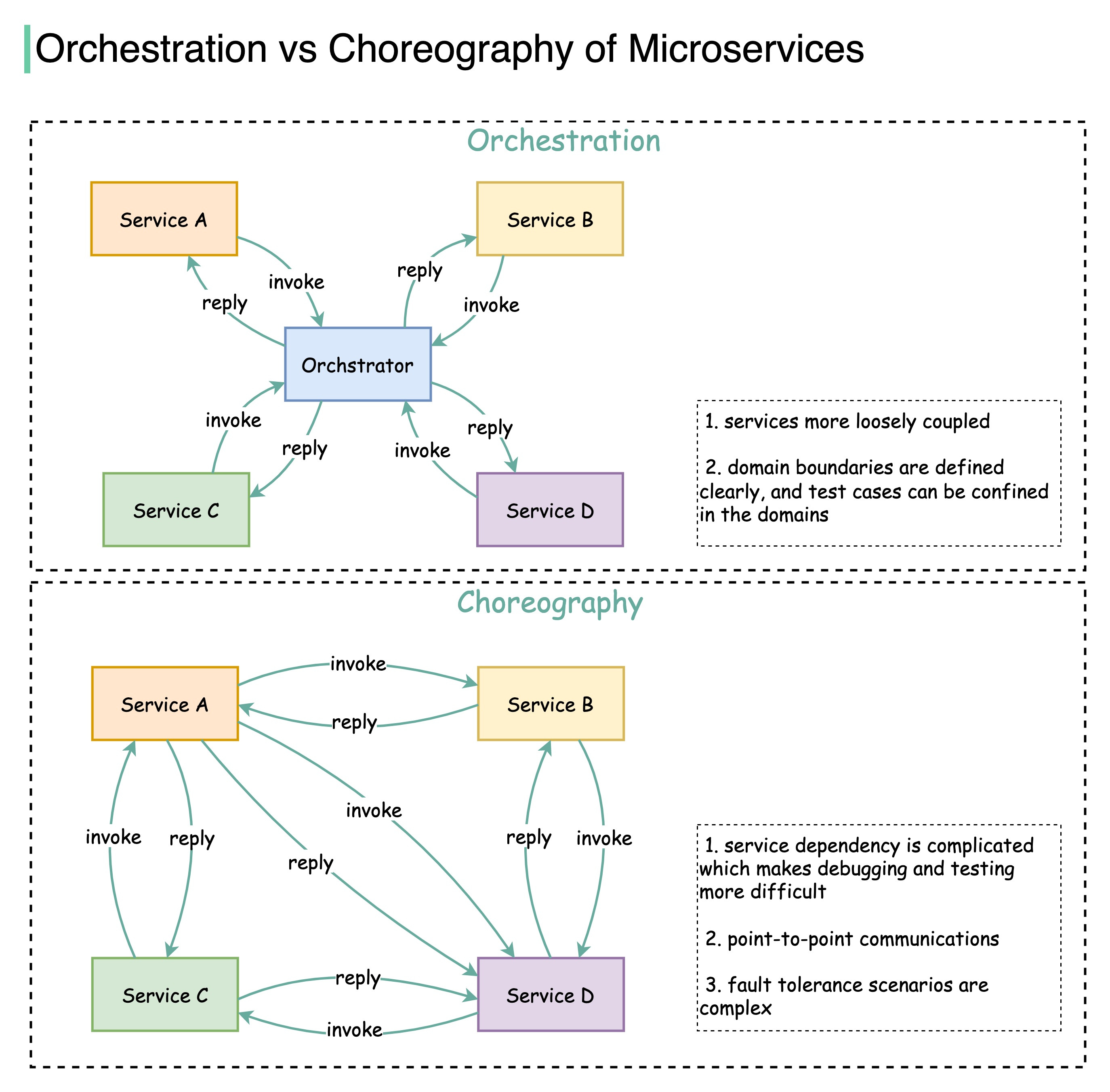
Credit: blog.bytebytego.com
Best Practices For Effective Collaboration
Microservices work best when they communicate and collaborate smoothly. Teams must follow some best practices. These practices help each service interact without issues. Clear communication ensures all services function together well.
Focus on simplicity and clarity. This makes it easier for teams to manage services. Proper collaboration leads to faster development and fewer mistakes. Here are key practices for effective collaboration among microservices.
Versioning
Versioning is crucial for microservices. Each service may change over time. Keeping track of these changes helps teams avoid conflicts. Using semantic versioning is a good approach. It clearly shows updates and compatibility.
Always document changes. This helps other teams understand updates. Use version numbers in API calls. This way, services can work together without breaking. Maintain backward compatibility whenever possible. This keeps existing users happy.
Continuous Integration/continuous Deployment (ci/cd)
CI/CD is essential for microservices collaboration. It allows teams to test and deploy updates quickly. With CI/CD, developers can catch issues early. This leads to a more stable system.
Automate the testing process. This reduces human error and speeds up releases. Set up pipelines for automated deployment. This ensures services are always up to date. Regular updates keep the system reliable and efficient.
Encourage teams to share their CI/CD practices. Learning from each other improves overall collaboration. A strong CI/CD culture leads to better teamwork and faster progress.
Credit: www.linkedin.com
Frequently Asked Questions
How Do Microservices Communicate With Each Other?
Microservices communicate using APIs, messaging, or event-driven methods. They send and receive data to work together effectively.
What Are The Benefits Of Microservices Collaboration?
Collaboration allows microservices to be flexible and scalable. They can be updated independently, improving overall system performance.
What Challenges Do Microservices Face In Interaction?
Microservices may struggle with network issues, data consistency, and managing different versions. These can affect their communication.
Conclusion
Microservices work together to create strong applications. They communicate through APIs and messages. This makes them flexible and easy to update. Each microservice handles its own tasks. This separation helps with performance and scaling. Teams can work on different parts at the same time.
Understanding how they interact is key to success. A well-designed microservices system leads to better software. Embracing this approach can improve your projects. Focus on collaboration for effective results. Keep exploring and learning about microservices. Your journey in software development can thrive with these insights.