Big O Notation is a key concept in computer science. It helps developers understand how efficient algorithms are.
In today’s fast-paced digital world, efficiency is crucial. Algorithms solve problems, and how well they do this matters. Big O Notation allows programmers to analyze the performance of their algorithms. It shows how the time or space needed changes as the input size grows.
This knowledge helps in choosing the right algorithm for the task. Whether you are a beginner or have some experience, understanding Big O Notation is essential. It can improve your coding skills and lead to better software. This guide will break down the basics of Big O Notation and how it can make your algorithms more efficient.
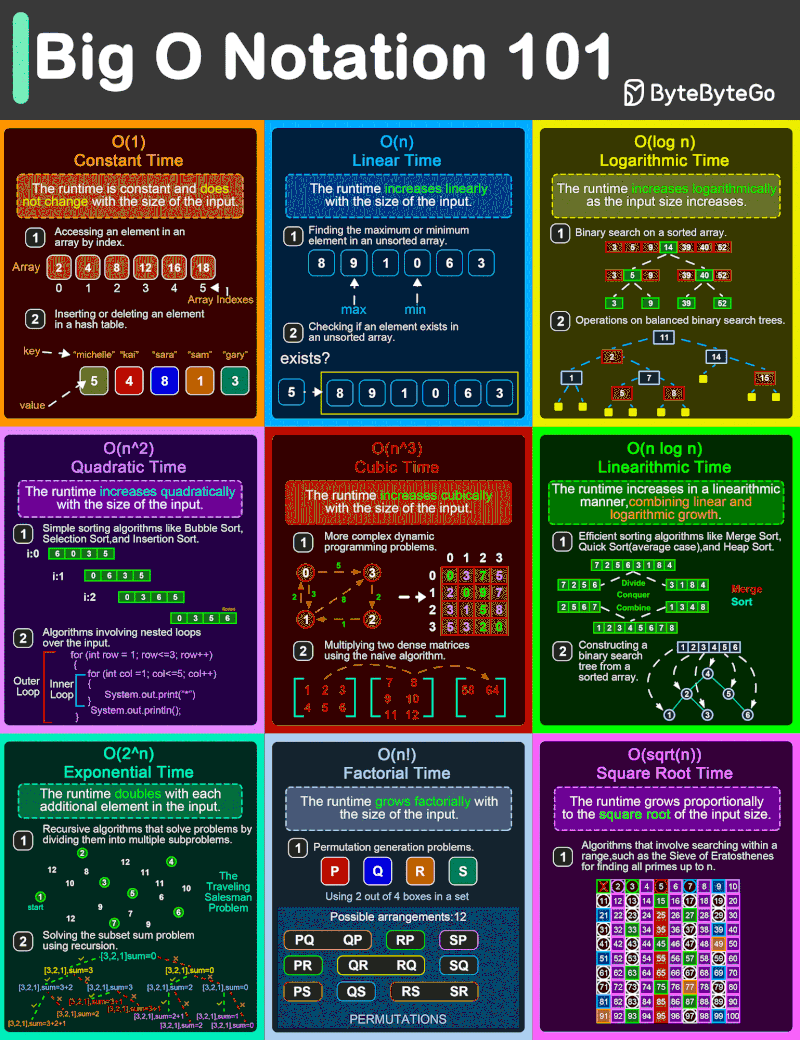
Credit: medium.com
Demystifying Big O Notation
Big O Notation is a way to measure algorithm efficiency. It helps us understand how algorithms perform as the input size grows. This concept can seem complex, but it is essential for writing efficient code. Let’s break it down into simpler terms.
The ABCs Of Algorithmic Complexity
Algorithmic complexity describes how long an algorithm takes to run. We focus on the input size, often denoted as “n.” Big O Notation uses symbols to express this complexity.
Common notations include O(1), O(n), O(n²), and O(log n). Each symbol represents a different growth rate. O(1) means constant time. The time stays the same, no matter how large n gets.
O(n) means linear time. As n increases, the time increases directly with it. O(n²) shows quadratic time. Time increases with the square of n. Finally, O(log n) indicates logarithmic time, which grows slower than linear time.
Visualizing Growth Rates In Big O
Visualising these growth rates helps grasp Big O Notation. Imagine a graph with input size on the x-axis and time on the y-axis. Each Big O notation will form a different curve.
The O(1) curve is flat, showing no growth. The O(n) line rises steadily. The O(n²) curve starts steep and rises quickly. The O(log n) line starts high but levels off.
This visualisation shows how different algorithms will perform. Choosing the right algorithm can save time and resources. Understanding these growth rates is key to writing better code.
Crucial Applications In Coding
Understanding Big O notation helps programmers write efficient code. It measures how an algorithm performs as the input size grows. This knowledge is vital in many coding tasks. Let’s explore two key applications: optimising search and sort functions and evaluating database queries.
Optimizing Search And Sort Functions
Search and sort functions are common in programming. They often need speed and efficiency. Using Big O Notation, programmers can choose the best algorithm for these tasks.
For example:
- Binary Search: Works on sorted data. It has a time complexity of O(log n).
- Linear Search: Checks each element one by one. Its time complexity is O(n).
Choosing the right search method can save time. A faster search means a better user experience.
Algorithm | Time Complexity |
---|---|
Binary Search | O(log n) |
Linear Search | O(n) |
Sorting algorithms also play a crucial role. Common sorting methods include:
- Quick Sort: Average case is O(n log n).
- Bubble Sort: The average case is O(n²).
Efficient sorting improves data handling. It speeds up data retrieval and processing.
Evaluating Database Queries
Database queries often involve large datasets. Here, Big O Notation helps assess performance. Efficient queries save time and resources.
Common SQL operations include:
- Select: Retrieves data. Performance varies based on indexes.
- Join: Combines tables. Can lead to complex queries.
Understanding the complexity of these operations is crucial. For instance:
Operation | Time Complexity |
---|---|
Simple Select | O(1) with index |
Join | O(n²) without index |
Optimising these queries can improve application performance. Use indexes wisely. They reduce the time taken to retrieve data.
Practical Tips For Algorithm Efficiency
Understanding algorithm efficiency is key. Practical tips help improve performance. These tips guide you to write better code. Let’s dive into two essential areas: refactoring code and benchmarking with Big O.
Refactoring Code For Performance
Refactoring makes your code cleaner and faster. Start by identifying slow parts of your code. Focus on loops and recursive functions. These often slow down performance.
Use efficient data structures. Arrays and linked lists serve different purposes. Choose wisely based on your needs. A hash table can speed up lookups.
Remove unnecessary calculations. Store results of expensive operations. This saves time when the same result is needed again.
Keep your code simple. Avoid complexity unless necessary. Simple code is easier to understand and maintain.
Benchmarking With Big O
Benchmarking helps you measure your algorithm’s efficiency. Use Big O notation to express time and space complexity. This notation shows how performance changes with input size.
Test your algorithms with different data sets. Observe how they perform as data grows. Understanding these patterns helps in improving your code.
Compare different algorithms using Big O. This allows you to choose the most efficient one for your task.
Remember to document your findings. Keeping track of performance helps in future projects. You can build on past experiences for better results.

Credit: blog.bytebytego.com
Credit: x.com
Frequently Asked Questions
What Is Big O Notation?
Big O Notation describes how an algorithm’s time or space requirements grow as the input size increases. It helps compare efficiency.
Why Is Big O Notation Important?
Big O Notation helps developers choose the best algorithms for tasks. It ensures programs run faster and use less memory.
How Do I Calculate Big O Notation?
To calculate Big O, analyze the algorithm’s loops and operations. Focus on the most significant factors affecting performance.
Conclusion
Understanding Big O notation is essential for writing efficient algorithms. It helps you analyze performance and predict how algorithms scale. This knowledge can improve your coding skills and make problem-solving easier. By using Big O, you can choose the best approach for your tasks.
Remember, efficiency matters in programming. Start applying these concepts today. Your future projects will benefit greatly from this knowledge. Embrace the power of Big O notation and enhance your coding journey.