Async and Await are tools in programming that help manage tasks. They make it easier to handle multiple operations at once without slowing down your program.
In today’s fast-paced digital world, speed and efficiency are crucial. Users expect apps to respond quickly, even while processing complex tasks. That’s where Async and Await come in. These programming concepts allow developers to write code that runs smoothly without freezing or lagging.
By using these tools, programmers can improve the user experience and make their applications more effective. Understanding Async and Await is essential for anyone looking to enhance their coding skills. In this blog post, I will explain what these terms mean and why they are beneficial for developers and users alike.

Credit: www.youtube.com
The Evolution Of Asynchronous Javascript
Asynchronous JavaScript has changed a lot over the years. It allows code to run without blocking other tasks. This is important for creating fast and responsive web applications.
Understanding how we got here helps us see why async and await are useful tools.
From Callbacks To Promises
Callbacks were the first way to handle asynchronous tasks. They let functions run after another function finishes. This method had its problems.
- Callback Hell: Deep nesting made code hard to read.
- Error Handling: Difficult to manage errors in multiple callbacks.
To solve these issues, Promises were introduced. Promises represent a value that may not be available yet. They provide a cleaner way to handle asynchronous code.
Feature | Callbacks | Promises |
---|---|---|
Readability | Hard to read | More readable |
Error Handling | Complex | Simpler |
Chaining | Not possible | Supports chaining |
The Arrival Of Async/await
With the rise of Promises, the need for even clearer syntax grew. Async and await were introduced in ES2017. They simplify writing asynchronous code.
Here is how they work:
- Async functions return a Promise automatically.
- Await pauses the execution until the Promise settles.
This makes the code look more like synchronous code. It is easier to follow. Here is a simple example:
async function fetchData() {
let response = await fetch('https://api.example.com/data');
let data = await response.json();
console.log(data);
}
Using async and await leads to cleaner code. It also improves error handling through try/catch blocks. This method is now the preferred way to handle asynchronous JavaScript.
Demystifying Async/await
Async and await are tools in JavaScript. They help manage tasks that take time. This includes tasks like fetching data from a server. Understanding them makes coding easier. It allows developers to write cleaner and more readable code.
The Basics Of Async Functions
Async functions are special. They return a promise. This promise can be either resolved or rejected. Here’s how they work:
- Async keyword: Use this keyword before a function.
- Returns a promise: The function will automatically return a promise.
- Readable code: Makes asynchronous code look like synchronous code.
Here is an example of an async function:
async function fetchData() {
let response = await fetch('https://api.example.com/data');
let data = await response.json();
return data;
}
How to Await Pauses Execution
Await is used inside async functions. It pauses the execution of the function. This allows other tasks to run.
Here’s how await works:
- Wait for a promise: The function will wait until the promise is resolved.
- Improves readability: Makes it easier to follow the code flow.
- Handles errors: You can use try-catch to handle errors effectively.
Example of using await:
async function getUser() {
try {
let user = await fetch('https://api.example.com/user');
let data = await user.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
Writing Cleaner Code With Async/await
Using Async and Await helps write clearer code. It makes your code easier to read. This is important for both new and experienced developers. You can focus on the logic without distractions.
Improving Readability
Async/Await makes code look more like regular code. It helps avoid complex structures. Here’s how:
- It allows you to write asynchronous code linearly.
- It reduces the need for nested functions.
- It makes error handling simpler.
Consider this example:
async function fetchData() {
try {
const data = await getData();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
This code is straightforward. You see the flow easily. You can understand what happens step by step.
Reducing Callback Hell
Callback hell occurs with multiple nested callbacks. It can make code hard to read and debug. Async/Await helps to avoid this issue. Instead of nesting, you can write flat code.
Here’s a comparison:
Callback Style | Async/Await Style |
---|---|
|
|
With Async/Await, the code is easier to follow. You can see each action clearly. This leads to fewer mistakes and faster debugging.

Credit: www.reddit.com
Error Handling In Async/await
Error handling is crucial in programming. It helps manage unexpected situations. In JavaScript, async/await makes error handling simpler. This method allows developers to write cleaner code. Let’s explore how to handle errors effectively with async functions.
Try/catch Blocks For Async Functions
Using try/catch blocks is a common way to handle errors. They help catch errors that occur in async functions. Here’s how it works:
- Wrap your async code in a try block.
- Use the catch block to handle errors.
Here’s an example:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching data:', error);
}
}
This code fetches data. If an error occurs, it logs the error message. This approach keeps your code organized and readable.
Common Pitfalls And How To Avoid Them
Many developers face issues with async/await. Here are some common pitfalls:
Pitfall | Solution |
---|---|
Not using try/catch | Always wrap async calls in try/catch. |
Forgetting to return promises | Ensure you return promises from async functions. |
Mixing async/await with then/catch | Stick to one style for better readability. |
Awareness of these pitfalls helps avoid bugs. Always test your async functions. This practice ensures your error handling works as intended.
Performance Implications
Async and await greatly affect how your code runs. They help manage long tasks without blocking the main thread. This means users can interact with your app smoothly. Understanding the performance implications is crucial for better coding.
Understanding Execution Flow
Execution flow changes with async and await. Normally, code runs in a straight line. With async, you can pause and wait for tasks to finish. This does not stop other code from running. It improves responsiveness.
When a task is awaited, the program yields control. This allows other tasks to run while waiting. The program does not freeze. Users continue to interact with the app. This is vital for user experience.
Best Practices For Efficient Code
Use async and await wisely to improve performance. Always mark functions that use await as async. This helps the program understand the flow. Keep tasks short and focused. Avoid long-running tasks within async functions.
Consider error handling. Use try-catch blocks to manage errors. This keeps your app stable. Limit the number of concurrent tasks. Too many can slow down performance.
Finally, test your code. Measure performance under different conditions. Optimize based on the results. Efficient use of async and await leads to better applications.
Async/await In Practice
Using Async and Await makes coding easier. They help manage tasks that take time. This includes tasks like fetching data or reading files. Here are some real-world examples to show how they work.
Real-world Examples
Imagine a website that shows weather data. Without Async/Await, the user waits for the data to load. This can be frustrating. With Async/Await, the site can show other content while fetching the weather data.
Another example is processing images. A program can start uploading an image. While uploading, it can also show a progress bar. Users see the progress without waiting. This keeps them engaged and happy.
Integrating With Apis And Databases
Integrating with APIs is common in apps. Async/Await makes it simple. For example, an app can get user data from an API. It does this without freezing the app. Users can continue interacting with the app.
Databases also benefit from Async/Await. A web app can query a database. While it waits for the response, the app remains responsive. Users can still navigate the app smoothly.
Using Async/Await leads to cleaner code. It makes debugging easier too. Developers can write code that is easy to read and maintain.
Tips And Tricks For Advanced Usage
Async and await can greatly improve your code. They simplify handling asynchronous tasks. Here are some tips for using them effectively.
Using Async/Await with Loops
Using async/await inside loops can be tricky. Each iteration may wait for the previous one. This can slow down your code. To speed things up, use Promise.all()
.
Wrap your async calls in an array. Then pass this array to Promise.all()
. This way, all promises run at the same time. It makes your code faster and more efficient.
Working With Multiple Promises
Handling multiple promises is easy with async/await. You can await each promise individually. This is simple but may slow your code.
To run promises in parallel, again use Promise.all()
. This method waits for all promises to settle. You get results in a single array. This method helps avoid nesting and keeps your code clean.
Always handle errors properly. Use try-catch blocks around your await calls. This prevents your application from crashing.
These tips will help you write better async code. Use them to improve performance and readability.
The Future Of Async/await
The future of Async/Await looks bright. This feature simplifies writing asynchronous code. Developers embrace it for its clarity and ease of use. It makes handling tasks more efficient.
As web applications grow, the need for better performance increases. Async/Await helps manage multiple tasks smoothly. It allows developers to write cleaner, more readable code. This will continue to matter as applications become more complex.
Emerging Patterns And Features
New patterns are emerging in Async/Await usage. Developers are finding creative ways to use it. For example, chaining multiple async functions can simplify workflows. This reduces complexity and improves code quality.
Other features, like error handling, are evolving. Using try/catch blocks with Async/Await makes error management easier. This results in more robust applications. Developers can focus on functionality, not just error tracking.
The Evolving Javascript Landscape
The JavaScript landscape is changing fast. New frameworks and libraries are adopting Async/Await. This trend enhances the development experience. It allows for more straightforward code, which is vital for teamwork.
As JavaScript evolves, Async/Await will remain important. It supports the shift toward modern programming practices. Developers are increasingly prioritizing readability and maintainability. Async/Await aligns perfectly with these goals.
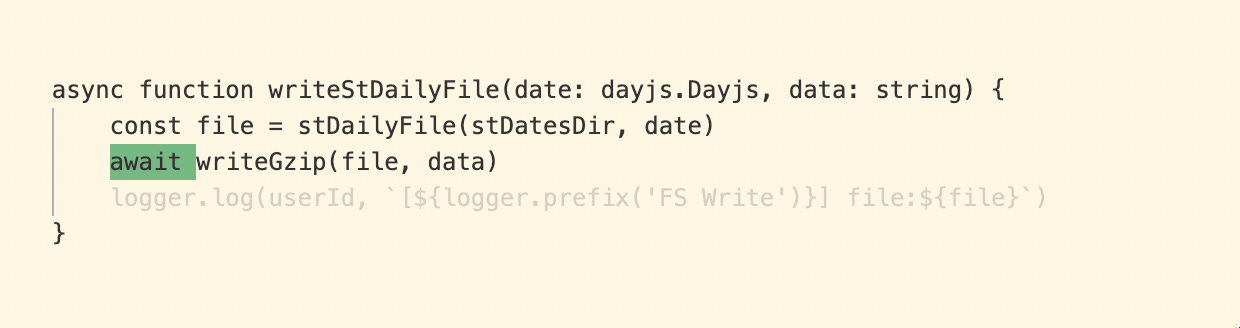
Credit: alexbezhan.substack.com
Frequently Asked Questions
What Is Async And Await In Programming?
Async and Await are tools in programming to handle tasks that take time. They help run code without blocking other tasks.
Why Should I Use Async And Await?
Using Async and Await makes your code easier to read and write. It helps improve performance by allowing tasks to run smoothly.
How Do Async And Await Improve Performance?
They let your program continue working while waiting for tasks to finish. This means users experience faster response times.
Conclusion
Async and await make coding easier. They help manage tasks without blocking. This means your program runs smoothly and quickly. Using these features can improve user experience. Errors become easier to handle too. Overall, they simplify complex code. With async and await, your code becomes cleaner and more efficient.
Many developers find them essential in modern programming. Embracing these tools can make your projects more successful. Start using async and await today. Your code will thank you.